출처: https://docs.oracle.com/javase/tutorial/java/javaOO/index.html
With the knowledge you now have of the basics of the Java programming language, you can learn to write your own classes. In this lesson, you will find information about defining your own classes, including declaring member variables, methods, and constructors.
You will learn to use your classes to create objects, and how to use the objects you create.
This lesson also covers nesting classes within other classes, and enumerations
Classes
The introduction to object-oriented concepts in the lesson titled Object-oriented Programming Concepts used a bicycle class as an example, with racing bikes, mountain bikes, and tandem bikes as subclasses. Here is sample code for a possible implementation of a Bicycle
class, to give you an overview of a class declaration. Subsequent sections of this lesson will back up and explain class declarations step by step. For the moment, don't concern yourself with the details.
public class Bicycle { // the Bicycle class has // three fields public int cadence; public int gear; public int speed; // the Bicycle class has // one constructor public Bicycle(int startCadence, int startSpeed, int startGear) { gear = startGear; cadence = startCadence; speed = startSpeed; } // the Bicycle class has // four methods public void setCadence(int newValue) { cadence = newValue; } public void setGear(int newValue) { gear = newValue; } public void applyBrake(int decrement) { speed -= decrement; } public void speedUp(int increment) { speed += increment; } }
A class declaration for a MountainBike
class that is a subclass of Bicycle
might look like this:
public class MountainBike extends Bicycle { // the MountainBike subclass has // one field public int seatHeight; // the MountainBike subclass has // one constructor public MountainBike(int startHeight, int startCadence, int startSpeed, int startGear) { super(startCadence, startSpeed, startGear); seatHeight = startHeight; } // the MountainBike subclass has // one method public void setHeight(int newValue) { seatHeight = newValue; } }
MountainBike
inherits all the fields and methods of Bicycle
and adds the field seatHeight
and a method to set it (mountain bikes have seats that can be moved up and down as the terrain demands).
Objects
A typical Java program creates many objects, which as you know, interact by invoking methods. Through these object interactions, a program can carry out various tasks, such as implementing a GUI, running an animation, or sending and receiving information over a network. Once an object has completed the work for which it was created, its resources are recycled for use by other objects.
Here's a small program, called CreateObjectDemo
, that creates three objects: one Point
object and two Rectangle
objects. You will need all three source files to compile this program.
public class CreateObjectDemo { public static void main(String[] args) { // Declare and create a point object and two rectangle objects. Point originOne = new Point(23, 94); Rectangle rectOne = new Rectangle(originOne, 100, 200); Rectangle rectTwo = new Rectangle(50, 100); // display rectOne's width, height, and area System.out.println("Width of rectOne: " + rectOne.width); System.out.println("Height of rectOne: " + rectOne.height); System.out.println("Area of rectOne: " + rectOne.getArea()); // set rectTwo's position rectTwo.origin = originOne; // display rectTwo's position System.out.println("X Position of rectTwo: " + rectTwo.origin.x); System.out.println("Y Position of rectTwo: " + rectTwo.origin.y); // move rectTwo and display its new position rectTwo.move(40, 72); System.out.println("X Position of rectTwo: " + rectTwo.origin.x); System.out.println("Y Position of rectTwo: " + rectTwo.origin.y); } }
This program creates, manipulates, and displays information about various objects. Here's the output:
Width of rectOne: 100 Height of rectOne: 200 Area of rectOne: 20000 X Position of rectTwo: 23 Y Position of rectTwo: 94 X Position of rectTwo: 40 Y Position of rectTwo: 72
The following three sections use the above example to describe the life cycle of an object within a program. From them, you will learn how to write code that creates and uses objects in your own programs. You will also learn how the system cleans up after an object when its life has ended.
Creating Objects
As you know, a class provides the blueprint for objects; you create an object from a class. Each of the following statements taken from the CreateObjectDemo
program creates an object and assigns it to a variable:
Point originOne = new Point(23, 94); Rectangle rectOne = new Rectangle(originOne, 100, 200); Rectangle rectTwo = new Rectangle(50, 100);
The first line creates an object of the Point
class, and the second and third lines each create an object of the Rectangle
class.
Each of these statements has three parts (discussed in detail below):
- Declaration: The code set in bold are all variable declarations that associate a variable name with an object type.
- Instantiation: The new keyword is a Java operator that creates the object.
- Initialization: The new operator is followed by a call to a constructor, which initializes the new object.
Declaring a Variable to Refer to an Object
Previously, you learned that to declare a variable, you write:
type name;
This notifies the compiler that you will use name to refer to data whose type is type. With a primitive variable, this declaration also reserves the proper amount of memory for the variable.
You can also declare a reference variable on its own line. For example:
Point originOne;
If you declare originOne
like this, its value will be undetermined until an object is actually created and assigned to it. Simply declaring a reference variable does not create an object. For that, you need to use the new
operator, as described in the next section. You must assign an object to originOne
before you use it in your code. Otherwise, you will get a compiler error.
A variable in this state, which currently references no object, can be illustrated as follows (the variable name, originOne
, plus a reference pointing to nothing):
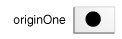
Instantiating a Class
The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory. The new operator also invokes the object constructor.
Note: The phrase "instantiating a class" means the same thing as "creating an object." When you create an object, you are creating an "instance" of a class, therefore "instantiating" a class.
The new operator requires a single, postfix argument: a call to a constructor. The name of the constructor provides the name of the class to instantiate.
The new operator returns a reference to the object it created. This reference is usually assigned to a variable of the appropriate type, like:
Point originOne = new Point(23, 94);
The reference returned by the new operator does not have to be assigned to a variable. It can also be used directly in an expression. For example:
int height = new Rectangle().height;
This statement will be discussed in the next section.
Initializing an Object
Here's the code for the Point class:
public class Point { public int x = 0; public int y = 0; //constructor public Point(int a, int b) { x = a; y = b; } }
This class contains a single constructor. You can recognize a constructor because its declaration uses the same name as the class and it has no return type. The constructor in the Point class takes two integer arguments, as declared by the code (int a, int b). The following statement provides 23 and 94 as values for those arguments:
Point originOne = new Point(23, 94);
The result of executing this statement can be illustrated in the next figure:
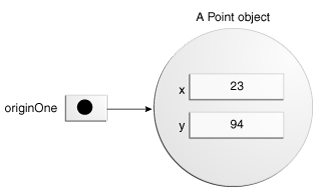
Here's the code for the Rectangle class, which contains four constructors:
public class Rectangle { public int width = 0; public int height = 0; public Point origin; // four constructors public Rectangle() { origin = new Point(0, 0); } public Rectangle(Point p) { origin = p; } public Rectangle(int w, int h) { origin = new Point(0, 0); width = w; height = h; } public Rectangle(Point p, int w, int h) { origin = p; width = w; height = h; } // a method for moving the rectangle public void move(int x, int y) { origin.x = x; origin.y = y; } // a method for computing the area of the rectangle public int getArea() { return width * height; } }
Each constructor lets you provide initial values for the rectangle's origin, width, and height, using both primitive and reference types. If a class has multiple constructors, they must have different signatures. The Java compiler differentiates the constructors based on the number and the type of the arguments. When the Java compiler encounters the following code, it knows to call the constructor in the Rectangle class that requires a Point argument followed by two integer arguments:
Rectangle rectOne = new Rectangle(originOne, 100, 200);
This calls one of Rectangle
's constructors that initializes origin
to originOne
. Also, the constructor sets width
to 100 and height
to 200. Now there are two references to the same Point object—an object can have multiple references to it, as shown in the next figure:
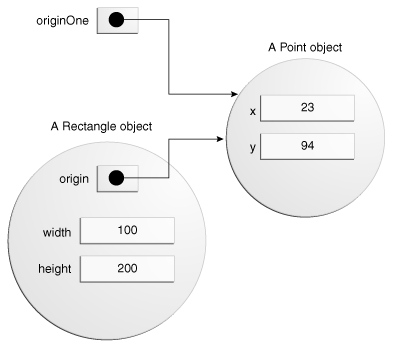
The following line of code calls the Rectangle
constructor that requires two integer arguments, which provide the initial values for width and height. If you inspect the code within the constructor, you will see that it creates a new Pointobject whose x and y values are initialized to 0:
Rectangle rectTwo = new Rectangle(50, 100);
The Rectangle constructor used in the following statement doesn't take any arguments, so it's called a no-argument constructor:
Rectangle rect = new Rectangle();
All classes have at least one constructor. If a class does not explicitly declare any, the Java compiler automatically provides a no-argument constructor, called the default constructor. This default constructor calls the class parent's no-argument constructor, or the Object
constructor if the class has no other parent. If the parent has no constructor (Object
does have one), the compiler will reject the program.
Using Objects
Once you've created an object, you probably want to use it for something. You may need to use the value of one of its fields, change one of its fields, or call one of its methods to perform an action.
Referencing an Object's Fields
Object fields are accessed by their name. You must use a name that is unambiguous.
You may use a simple name for a field within its own class. For example, we can add a statement within the Rectangle
class that prints the width
and height
:
System.out.println("Width and height are: " + width + ", " + height);
In this case, width
and height
are simple names.
Code that is outside the object's class must use an object reference or expression, followed by the dot (.) operator, followed by a simple field name, as in:
objectReference.fieldName
For example, the code in the CreateObjectDemo class is outside the code for the Rectangle class. So to refer to the origin, width, and height fields within the Rectangle object named rectOne, the CreateObjectDemo class must use the namesrectOne.origin, rectOne.width, and rectOne.height, respectively. The program uses two of these names to display the width and the height of rectOne:
System.out.println("Width of rectOne: " + rectOne.width); System.out.println("Height of rectOne: " + rectOne.height);
Attempting to use the simple names width and height from the code in the CreateObjectDemo class doesn't make sense — those fields exist only within an object — and results in a compiler error.
Later, the program uses similar code to display information about rectTwo. Objects of the same type have their own copy of the same instance fields. Thus, each Rectangle object has fields named origin, width, and height. When you access an instance field through an object reference, you reference that particular object's field. The two objects rectOne and rectTwo in the CreateObjectDemo program have different origin, width, and height fields.
To access a field, you can use a named reference to an object, as in the previous examples, or you can use any expression that returns an object reference. Recall that the new operator returns a reference to an object. So you could use the value returned from new to access a new object's fields:
int height = new Rectangle().height;
This statement creates a new Rectangle object and immediately gets its height. In essence, the statement calculates the default height of a Rectangle. Note that after this statement has been executed, the program no longer has a reference to the created Rectangle, because the program never stored the reference anywhere. The object is unreferenced, and its resources are free to be recycled by the Java Virtual Machine.
Calling an Object's Methods
You also use an object reference to invoke an object's method. You append the method's simple name to the object reference, with an intervening dot operator (.). Also, you provide, within enclosing parentheses, any arguments to the method. If the method does not require any arguments, use empty parentheses.
objectReference.methodName(argumentList);
or:
objectReference.methodName();
The Rectangle class has two methods: getArea() to compute the rectangle's area and move() to change the rectangle's origin. Here's the CreateObjectDemo code that invokes these two methods:
System.out.println("Area of rectOne: " + rectOne.getArea()); ... rectTwo.move(40, 72);
The first statement invokes rectOne's getArea()
method and displays the results. The second line moves rectTwo because the move() method assigns new values to the object's origin.x and origin.y.
As with instance fields, objectReference must be a reference to an object. You can use a variable name, but you also can use any expression that returns an object reference. The new operator returns an object reference, so you can use the value returned from new to invoke a new object's methods:
new Rectangle(100, 50).getArea()
The expression new Rectangle(100, 50) returns an object reference that refers to a Rectangle object. As shown, you can use the dot notation to invoke the new Rectangle's getArea() method to compute the area of the new rectangle.
Some methods, such as getArea(), return a value. For methods that return a value, you can use the method invocation in expressions. You can assign the return value to a variable, use it to make decisions, or control a loop. This code assigns the value returned by getArea() to the variable areaOfRectangle
:
int areaOfRectangle = new Rectangle(100, 50).getArea();
Remember, invoking a method on a particular object is the same as sending a message to that object. In this case, the object that getArea() is invoked on is the rectangle returned by the constructor.
The Garbage Collector
Some object-oriented languages require that you keep track of all the objects you create and that you explicitly destroy them when they are no longer needed. Managing memory explicitly is tedious and error-prone. The Java platform allows you to create as many objects as you want (limited, of course, by what your system can handle), and you don't have to worry about destroying them. The Java runtime environment deletes objects when it determines that they are no longer being used. This process is called garbage collection.
An object is eligible for garbage collection when there are no more references to that object. References that are held in a variable are usually dropped when the variable goes out of scope. Or, you can explicitly drop an object reference by setting the variable to the special value null. Remember that a program can have multiple references to the same object; all references to an object must be dropped before the object is eligible for garbage collection.
The Java runtime environment has a garbage collector that periodically frees the memory used by objects that are no longer referenced. The garbage collector does its job automatically when it determines that the time is right.
More on Classes
This section covers more aspects of classes that depend on using object references and the dot
operator that you learned about in the preceding sections on objects:
- Returning values from methods.
- The
this
keyword. - Class vs. instance members.
- Access control.
Nested Classes
The Java programming language allows you to define a class within another class. Such a class is called a nested class and is illustrated here:
class OuterClass { ... class NestedClass { ... } }
Terminology: Nested classes are divided into two categories: static and non-static. Nested classes that are declared
static
are called static nested classes. Non-static nested classes are called inner classes.class OuterClass { ... static class StaticNestedClass { ... } class InnerClass { ... } }
A nested class is a member of its enclosing class. Non-static nested classes (inner classes) have access to other members of the enclosing class, even if they are declared private. Static nested classes do not have access to other members of the enclosing class. As a member of the OuterClass
, a nested class can be declared private
, public
, protected
, or package private. (Recall that outer classes can only be declared public
or package private.)
Why Use Nested Classes?
Compelling reasons for using nested classes include the following:
It is a way of logically grouping classes that are only used in one place: If a class is useful to only one other class, then it is logical to embed it in that class and keep the two together. Nesting such "helper classes" makes their package more streamlined.
It increases encapsulation: Consider two top-level classes, A and B, where B needs access to members of A that would otherwise be declared
private
. By hiding class B within class A, A's members can be declared private and B can access them. In addition, B itself can be hidden from the outside world.It can lead to more readable and maintainable code: Nesting small classes within top-level classes places the code closer to where it is used.
Static Nested Classes
As with class methods and variables, a static nested class is associated with its outer class. And like static class methods, a static nested class cannot refer directly to instance variables or methods defined in its enclosing class: it can use them only through an object reference.
Note: A static nested class interacts with the instance members of its outer class (and other classes) just like any other top-level class. In effect, a static nested class is behaviorally a top-level class that has been nested in another top-level class for packaging convenience.
Static nested classes are accessed using the enclosing class name:
OuterClass.StaticNestedClass
For example, to create an object for the static nested class, use this syntax:
OuterClass.StaticNestedClass nestedObject = new OuterClass.StaticNestedClass();
Inner Classes
As with instance methods and variables, an inner class is associated with an instance of its enclosing class and has direct access to that object's methods and fields. Also, because an inner class is associated with an instance, it cannot define any static members itself.
Objects that are instances of an inner class exist within an instance of the outer class. Consider the following classes:
class OuterClass { ... class InnerClass { ... } }
An instance of InnerClass
can exist only within an instance of OuterClass
and has direct access to the methods and fields of its enclosing instance.
To instantiate an inner class, you must first instantiate the outer class. Then, create the inner object within the outer object with this syntax:
OuterClass.InnerClass innerObject = outerObject.new InnerClass();
There are two special kinds of inner classes: local classes and anonymous classes.
Shadowing
If a declaration of a type (such as a member variable or a parameter name) in a particular scope (such as an inner class or a method definition) has the same name as another declaration in the enclosing scope, then the declarationshadows the declaration of the enclosing scope. You cannot refer to a shadowed declaration by its name alone. The following example, ShadowTest
, demonstrates this:
public class ShadowTest { public int x = 0; class FirstLevel { public int x = 1; void methodInFirstLevel(int x) { System.out.println("x = " + x); System.out.println("this.x = " + this.x); System.out.println("ShadowTest.this.x = " + ShadowTest.this.x); } } public static void main(String... args) { ShadowTest st = new ShadowTest(); ShadowTest.FirstLevel fl = st.new FirstLevel(); fl.methodInFirstLevel(23); } }
The following is the output of this example:
x = 23 this.x = 1 ShadowTest.this.x = 0
This example defines three variables named x
: the member variable of the class ShadowTest
, the member variable of the inner class FirstLevel
, and the parameter in the method methodInFirstLevel
. The variable x
defined as a parameter of the method methodInFirstLevel
shadows the variable of the inner class FirstLevel
. Consequently, when you use the variable x
in the method methodInFirstLevel
, it refers to the method parameter. To refer to the member variable of the inner class FirstLevel
, use the keyword this
to represent the enclosing scope:
System.out.println("this.x = " + this.x);
Refer to member variables that enclose larger scopes by the class name to which they belong. For example, the following statement accesses the member variable of the class ShadowTest
from the method methodInFirstLevel
:
System.out.println("ShadowTest.this.x = " + ShadowTest.this.x);
Serialization
Serialization of inner classes, including local and anonymous classes, is strongly discouraged. When the Java compiler compiles certain constructs, such as inner classes, it creates synthetic constructs; these are classes, methods, fields, and other constructs that do not have a corresponding construct in the source code. Synthetic constructs enable Java compilers to implement new Java language features without changes to the JVM. However, synthetic constructs can vary among different Java compiler implementations, which means that .class
files can vary among different implementations as well. Consequently, you may have compatibility issues if you serialize an inner class and then deserialize it with a different JRE implementation. See the section Implicit and Synthetic Parameters in the section Obtaining Names of Method Parameters for more information about the synthetic constructs generated when an inner class is compiled.
Inner Class Example
To see an inner class in use, first consider an array. In the following example, you create an array, fill it with integer values, and then output only values of even indices of the array in ascending order.
The DataStructure.java
example that follows consists of:
- The
DataStructure
outer class, which includes a constructor to create an instance ofDataStructure
containing an array filled with consecutive integer values (0, 1, 2, 3, and so on) and a method that prints elements of the array that have an even index value. - The
EvenIterator
inner class, which implements theDataStructureIterator
interface, which extends theIterator
<
Integer
>
interface. Iterators are used to step through a data structure and typically have methods to test for the last element, retrieve the current element, and move to the next element. - A
main
method that instantiates aDataStructure
object (ds
), then invokes theprintEven
method to print elements of the arrayarrayOfInts
that have an even index value.
public class DataStructure { // Create an array private final static int SIZE = 15; private int[] arrayOfInts = new int[SIZE]; public DataStructure() { // fill the array with ascending integer values for (int i = 0; i < SIZE; i++) { arrayOfInts[i] = i; } } public void printEven() { // Print out values of even indices of the array DataStructureIterator iterator = this.new EvenIterator(); while (iterator.hasNext()) { System.out.print(iterator.next() + " "); } System.out.println(); } interface DataStructureIterator extends java.util.Iterator<Integer> { } // Inner class implements the DataStructureIterator interface, // which extends the Iterator<Integer> interface private class EvenIterator implements DataStructureIterator { // Start stepping through the array from the beginning private int nextIndex = 0; public boolean hasNext() { // Check if the current element is the last in the array return (nextIndex <= SIZE - 1); } public Integer next() { // Record a value of an even index of the array Integer retValue = Integer.valueOf(arrayOfInts[nextIndex]); // Get the next even element nextIndex += 2; return retValue; } } public static void main(String s[]) { // Fill the array with integer values and print out only // values of even indices DataStructure ds = new DataStructure(); ds.printEven(); } }
The output is:
0 2 4 6 8 10 12 14
Note that the EvenIterator
class refers directly to the arrayOfInts
instance variable of the DataStructure
object.
You can use inner classes to implement helper classes such as the one shown in the this example. To handle user interface events, you must know how to use inner classes, because the event-handling mechanism makes extensive use of them.
Local and Anonymous Classes
There are two additional types of inner classes. You can declare an inner class within the body of a method. These classes are known as local classes. You can also declare an inner class within the body of a method without naming the class. These classes are known as anonymous classes.
Modifiers
You can use the same modifiers for inner classes that you use for other members of the outer class. For example, you can use the access specifiers private
, public
, and protected
to restrict access to inner classes, just as you use them to restrict access do to other class members.
ㄴ
ㄴ
ㄴ
ㄴ
ㄴ
'Programming & Network > Java' 카테고리의 다른 글
JSTL items length size item index 값 확인 - JSTL 강좌 LoopTagStatus varStatus (0) | 2016.06.17 |
---|---|
(Mac Eclipse) shortcut (0) | 2016.03.13 |
Language Basics (0) | 2016.03.12 |
About Java (0) | 2016.03.12 |
유용한 사이트들 모음 (0) | 2016.03.08 |